Run the Emulator directly in Android Studio
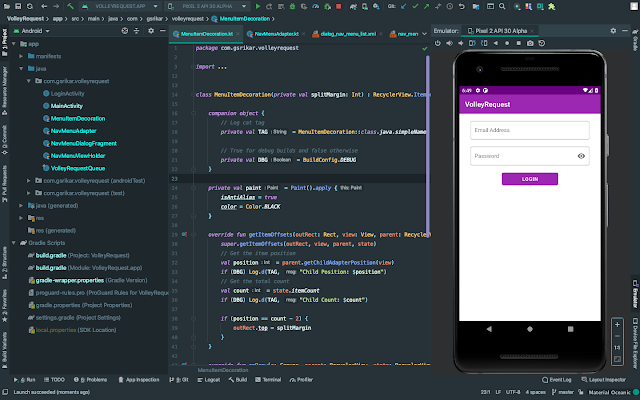
In Android Studio 4.1, a minor feature is released to reduce the screen (or window) switching between Android Studio and the Emulator. Using this feature, we can now run the Emulator directly inside the Android Studio. We can make the changes to the code and see the changes reflect in the emulator in the same window similar to layout XML and its preview. To achieve this, open the Preferences window and select the Emulator menu item under the Tools expandable menu. Here we can check the option Launch in a tool window and click OK . Now run the Emulator to see it inside the Android Studio window.